Same Origin Policy (SOP) - Lab 2 & 3
Intro
In Lab 1 we talked about SOP rules and why we need SOP. Today, we deep dive into two of SOP rules which are embedding and reading.
Let’s get started 🚀
⚡ Lab 2 : cross-origin embedding: allowed
Embedding resources, such as images/videos/Javascripts from a different origin, does not violate SOP and they should be allowed.
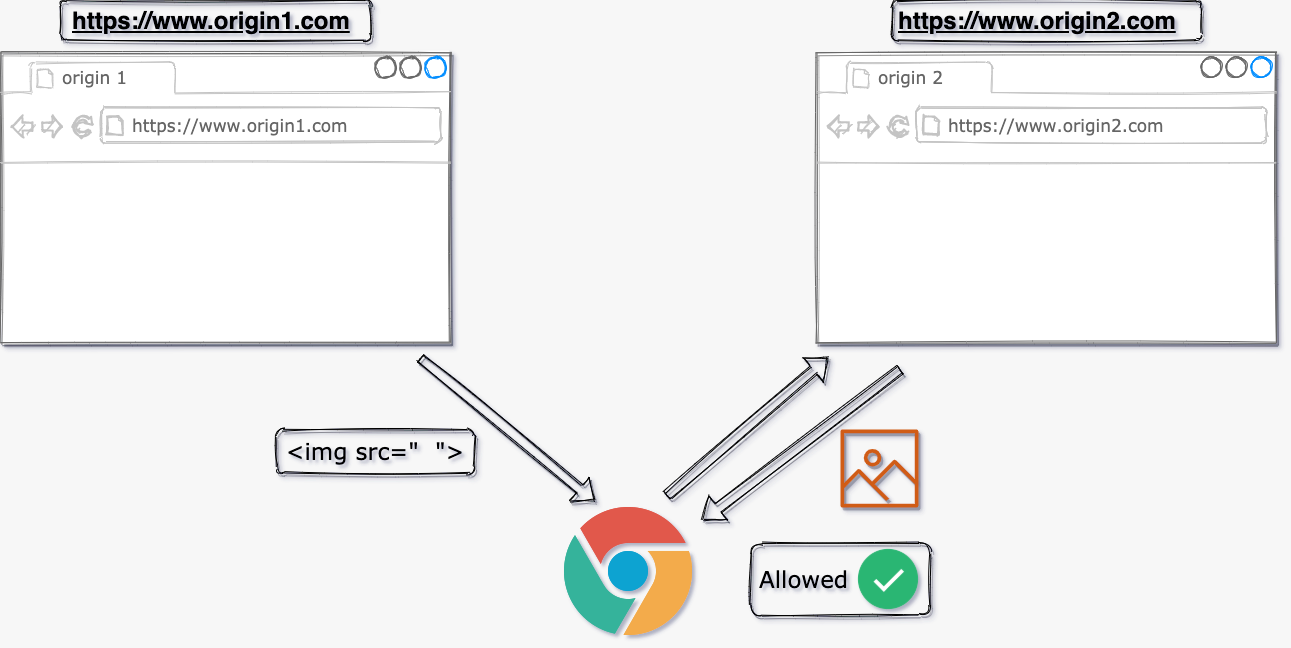
Let’s confirm this rule by ourselves 😉
-
Start network monitor in your browser developer tool (I will be using Firefox)
-
Open this web page
-
Notice that we could load (embed) some resources like course_image.png successfully.
There are also some JS files have been loaded successfully (readJSON.js and PrintSecureCookie.js).
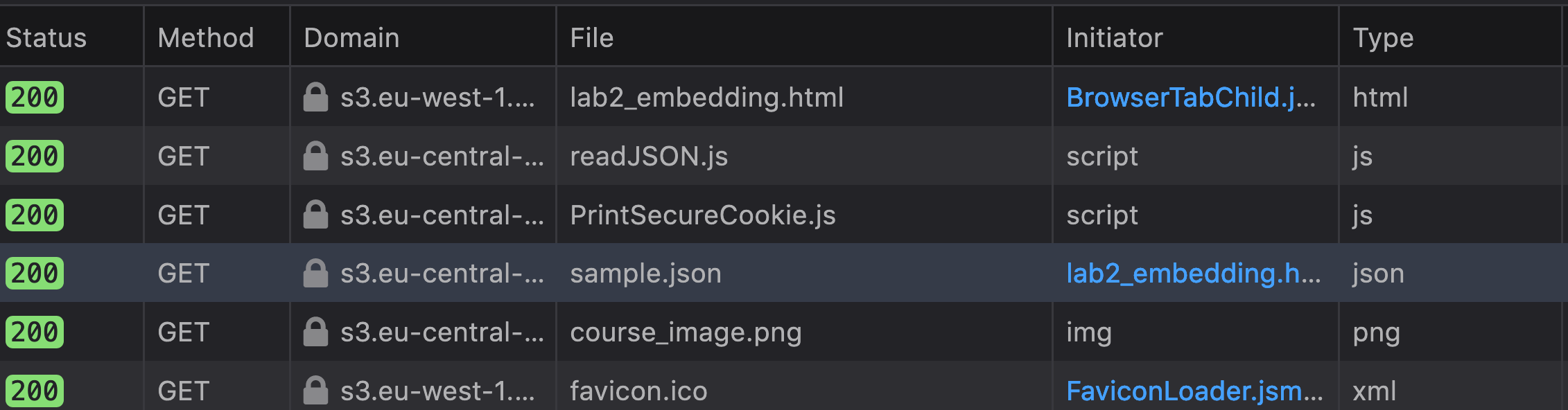
These resources are loaded from origin
https://s3.eu-central-1.amazonaws.com
Into origin
https://s3.eu-west-1.amazonaws.com
so what we saw here 👆 confirms that
Embedding resources, such as images/videos/Javascripts from a different origin, does not violate SOP
- Right now, open the console
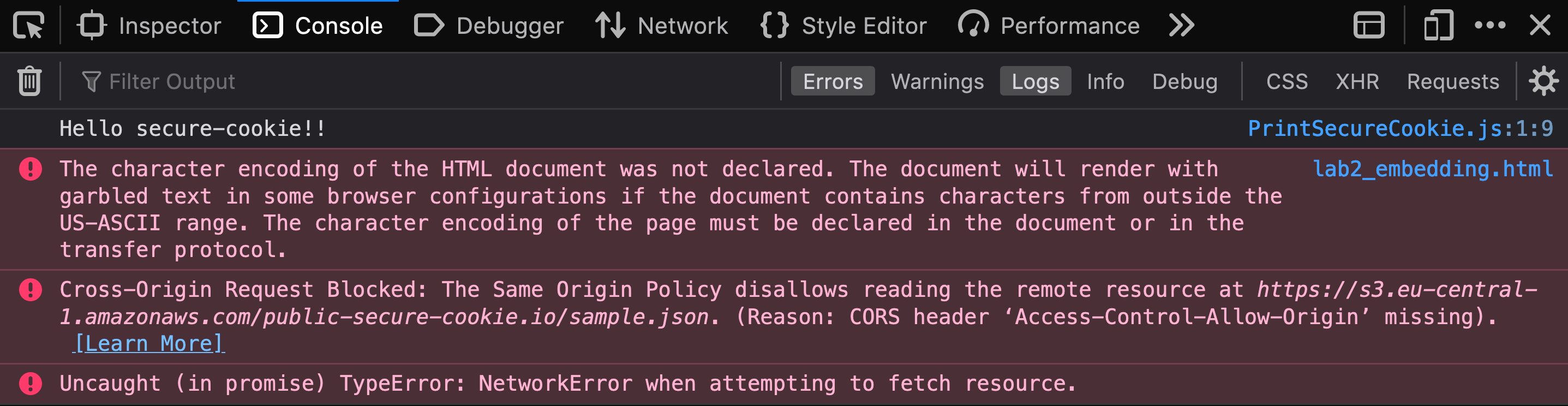
and notice that the execution of a JS (PrintSecureCookie.js) file logs a message into the console.
Hello secure-cookie!!
On the other hand, the execution of JS (readJSON.js) is making a problem and causing a warning msg
Cross-Origin Request Blocked: The Same Origin Policy disallows reading the remote resource at
https://s3.eu-central-1.amazonaws.com/public-secure-cookie.io/sample.json
(Reason: CORS header ‘Access-Control-Allow-Origin’ missing).
What is this message? and why did this happen?
While SOP allows embedding resources, it does not allow reading resources.
Check out lab 3 to find out answers!
⚡ Lab 3 : cross-origin read : disallowed
In figure 1
we saw that we got 200 STATUS
on fetching all the web resources like images and Javascripts.
On the other hand, in figure 2 we saw that same origin policy
blocked a JS (readJSON.js
) from reading a resource (sample.json
).
Let’s talk about the difference between reading and embedding.
The website origin is
https://s3.eu-west-1.amazonaws.com
And the html was loading a JS (readJSON.js
) from origin (https://s3.eu-central-1.amazonaws.com
)
using <script>
tag 👇.
<script src="https://s3.eu-central-1.amazonaws.com/public-secure-cookie.io/readJSON.js"></script>
As we said, SOP allows embedding cross-origin resources. So fetching
the JS (readJSON.js
) code itself happened without any issue. That explains 200 ✅ status .
Now executing the JS (readJSON.js
) code after loading it, is a different story 😅.
Having a look on the source code of the JS (readJSON.js
) file, tells us that it:-
url = "https://s3.eu-central-1.amazonaws.com/
public-secure-cookie.io/sample.json"
fetch(url,{method: 'GET'}).then(response =>
response.json()).then(function (data) {
console.log(data)
});
- GET sample.json file from
https://s3.eu-central-1.amazonaws.com
origin. This is allowed ✅ by SOP and therefore you see200 status
code . - Reads the content of the json file and log it to the console. This is blocked ❌ by SOP and therefore you see the exception in the console.
Even though the JS (readJSON.js
) was loaded from origin:-
https://s3.eu-central-1.amazonaws.com
Once it gets loaded to a page, it does NOT belong to the hosting origin anymore, it now belongs to the page origin:-
https://s3.eu-west-1.amazonaws.com
So the JS (readJSON.js
) is actually making a cross-origin HTTP request,
to fetch sample.json from https://s3.eu-central-1.amazonaws.com
.
The browser allows to fetch sample.json but it disallow the JS (readJSON.js
) from reading it due to SOP reading rule.
Then, how to read cross origin resources❓
We are living in the age of microservices. Many services and APIs for the same app are deployed in different domains and that means different origins.
In order to read cross-origin resources, we need to use Cross Origin Resource Sharing (CORS) policy.
CORS is a policy that allows a list of pre-defined origin(s) to read a resource(s).
We will talk about CORS in separate article.