Cross Origin Resource Sharing - CORS
Intro
In same origin policy lab 3 we saw that Javascript (JS) can’t read cross-origin resources.
That is a problem becasue many services and APIs for the same app are deployed on different domains and that means different origins.
Meaning, due to same origin policy, this domain
service1.example.com
can not read resources from this domain
servce2.example.com
Cross Origin Resource Sharing (CORS) can solve this.
What is Cross Origin Resource Sharing (CORS)❓
It’s a way to make an exception for same-origin policy. With CORS, servce2.example.com
can make exception and allow service1.example.com
to read its resources.
How to configure it❓
Via HTTP headers.
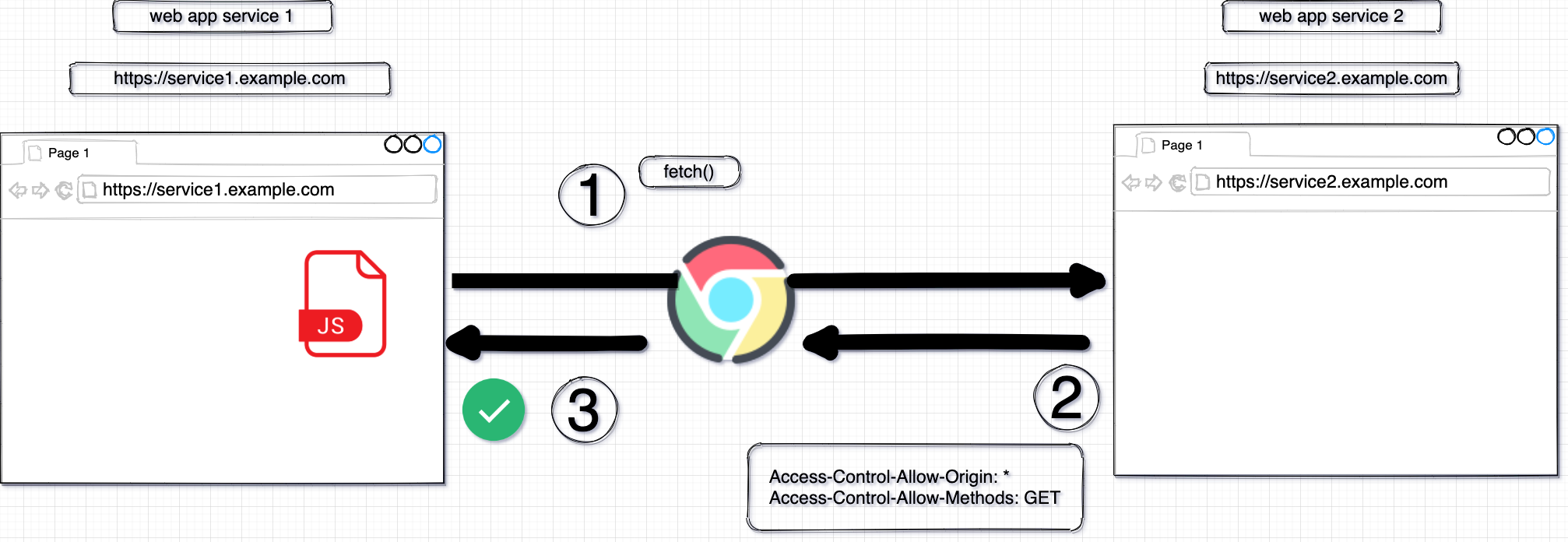
-
When a JS in origin
service1.example.com
wants to read resource from origin
service2.example.com
-
API endpoint for
service2.example.com
can return HTTP headers to allowservice1.example.com
origin to read a resource. -
Once the browser sees that
service1.example.com
origin is allowed, then it will allow the JS to read the response.
⚡Quick Lab
-
Fire up your browser’s console.
-
Paste this JS snippet and hit enter, it will :-
url = "https://s3.eu-central-1.amazonaws.com/cors-enabled-secure-cookie.io/sample.json"
fetch(url,{method: 'GET'}).then(response =>
response.json()).then(function (data) {
console.log(data)
});
- GET sample.json file from
https://s3.eu-central-1.amazonaws.com
origin. - Reads the content of the json file and log it to the console.
Jump to the network tab and inspect the response HTTP headers.
Access-Control-Allow-Origin: *
Access-Control-Allow-Methods: GET
Server: AmazonS3
-
The endpoint (AWS S3) allows ANY (*) origin to read its resources using GET method.
-
The browser will validate the request (origin and HTTP method) against the returned policy (in the HTTP response header). If origin and method are allowed then the browser will allow JS to read the content of the resource.
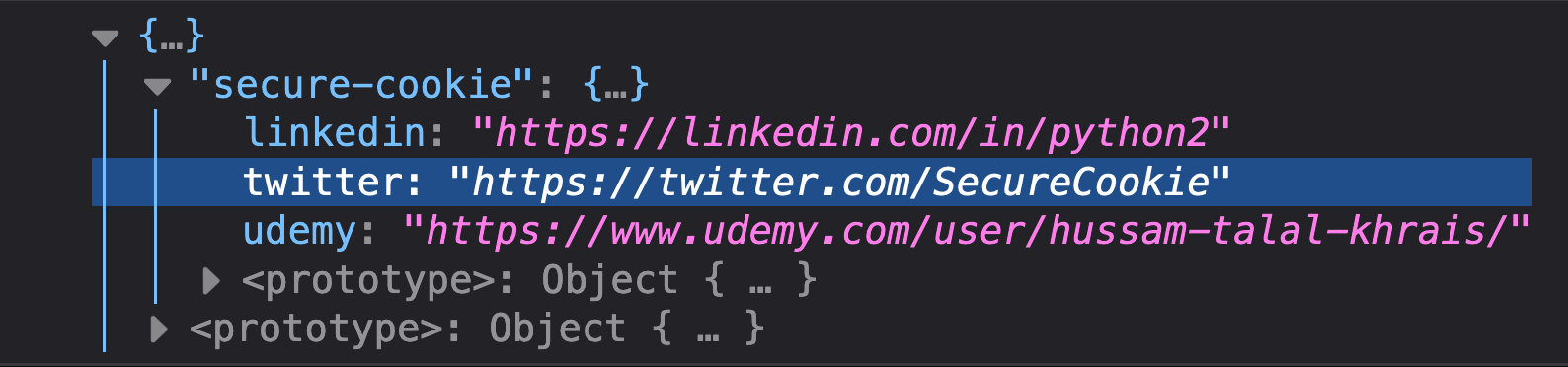
AWS S3 configuration
CORS policy could be configured on:-
- backend app (Python flask, Django, PHP)
- API service (like AWS API gateway)
- storage like AWS S3
In this lab I used AWS S3 bucket (cors-enabled-secure-cookie.io). Here’s the CORS policy that is attached to the bucket.
{
"AllowedHeaders": [
"*"
],
"AllowedMethods": [
"GET"
],
"AllowedOrigins": [
"*"
]
}